Have you ever wondered how a car’s engine knows exactly how much fuel to inject based on the speed you’re going, or how a calculator can instantly perform complex operations? The secret lies in the powerful concept of functions, and today, we’re diving deeper into their core components: parameters and return values.

Image: www.dreamstime.com
Imagine functions as miniature, specialized machines, taking inputs (parameters) and producing outputs (return values). This lesson will equip you with the knowledge to understand how to send information into a function, have it perform its task, and retrieve the calculated result. Mastering this skill is crucial for building efficient and reusable code, vital for any programmer.
Understanding Parameters: The Ingredients of Your Function
Parameters act like ingredients in a recipe. When you call a function, you pass in specific values – the parameters – that the function then uses to perform its calculations. For example, a function designed to calculate the area of a rectangle would expect two parameters: the length and width.
Example:
Let’s illustrate this with a simple example in Python:
def calculate_area(length, width):
area = length * width
return area
# Calling the function with specific parameters
rectangle_length = 5
rectangle_width = 10
area = calculate_area(rectangle_length, rectangle_width)
print(f"The area of the rectangle is: area")
In this example, the `calculate_area` function receives two parameters: `length` and `width`. It then uses these values to calculate the area and returns the result. When you call the function with specific lengths and widths, it produces the corresponding area.
Return Values: The Function’s Output
Return values are the results of a function’s work, the product of the process. Just like a chef presents you with a delicious dish after using the ingredients, a function returns a value based on the parameters it received. This value can then be used in other parts of your code or displayed as output.
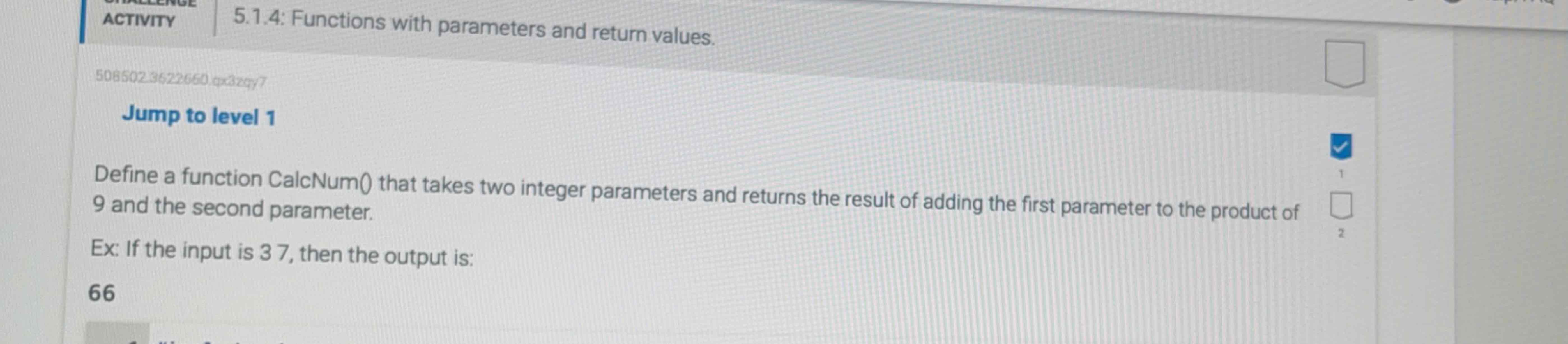
Image: www.chegg.com
Example:
Continuing with the area calculation example, the function `calculate_area` returns the calculated `area` value:
def calculate_area(length, width):
area = length * width
return area
# Call the function and store the returned value
area = calculate_area(5, 10)
# Use the returned value
print(f"The area of the rectangle is: area")
Here, the `calculate_area` function returns the calculated area, which is then stored in the variable `area`. This value is then used to display the final result to the user.
Putting Parameters and Return Values to Work
Now that you understand the basic concepts, let’s explore how parameters and return values are used to create powerful and flexible code. Imagine building a program that calculates the average of a list of numbers:
def calculate_average(numbers):
total = 0
for number in numbers:
total += number
average = total / len(numbers)
return average
# Example usage
numbers = [10, 20, 30, 40]
average = calculate_average(numbers)
print(f"The average of the numbers is: average")
In this example, the `calculate_average` function takes a single parameter `numbers` – a list of numbers. It then calculates the average of those numbers and returns the final value. Notice how the function can be used with any list of numbers, making it reusable and efficient.
Types of Parameters and Return Values
Beyond simple numeric values, functions can work with different data types as parameters and return values. Let’s explore some key types:
1. String Parameters and Return Values:
You can pass strings as parameters, manipulate them within a function, and return modified strings. For example, a function could take a string and capitalize the first letter of each word.
2. Lists and Arrays:
Functions can receive lists or arrays as parameters, process the elements, and return modified lists or new lists based on specific operations.
3. Dictionaries:
Functions can work with dictionaries, retrieving information, updating values, or even returning new dictionaries based on given criteria.
Real-World Applications
Parameters and return values are not just theoretical concepts; they power countless applications in everyday life. Here are a few examples:
- Online Shopping: When you add items to your cart, a function might take your shopping cart as a parameter, process the item details, and return an updated cart with the new item added.
- Navigation Apps: When you request directions, a function might take your current location and destination as parameters, process them using map data, and return the optimal route with estimated travel time.
- Social Media Platforms: Posting a status update on a social media platform might involve a function taking the text of your post as a parameter, adding timestamp and other details, and then returning the post to be displayed on your profile.
Important Considerations
While parameters and return values are powerful tools, there are some important points to keep in mind:
- Parameter Validation: It’s essential to validate incoming parameters to prevent errors. Check if they are of the correct data type and within an expected range.
- Return Value Handling: Ensure you handle the returned value correctly in your code, either storing it in a variable or directly using it for further processing.
- Function Documentation: Clearly document the parameters your function expects, the type of data it processes, and the return value it produces. This makes your code easier to understand and maintain.
Lesson 4 Parameters And Return Make
Conclusion
Understanding parameters and return values is fundamental to mastering functional programming. By effectively defining parameters, performing computations based on those inputs, and returning meaningful results, you will lay the foundation for building robust and efficient code. Remember to utilize various data types, validate your parameters, and clearly document your functions. Armed with this knowledge, you can unleash the full potential of functions in your coding journey!